Restful Routing
Published by
sanya sanya
RESTful routing in Express.js refers to designing and implementing routes in an Express.js application that follow the principles of REST
(Representational State Transfer). It involves mapping HTTP methods and URLs to specific actions or operations on resources.
In RESTful routing
with Express.js, you assign different HTTP methods to routes that represent different actions on resources. The routes are structured in a way that reflects the hierarchy and relationship between resources.
CRUD Operations
CRUD Operations are essential database operations that allow users to Create, Read, Update, and Delete data records.
- Create: Insert new data records into the database.
- Read: Retrieve and view existing data records from the database.
- Update: Modify and edit existing data records in the database.
- Delete: Remove and erase unwanted data records from the database.
Restful Routes
Some commonly used RESTful routes
and their corresponding HTTP methods are mentioned below -
- GET /resource - Retrieves a collection of resources.
- GET /resource/id - Retrieves a specific resource by its identifier.
- POST /resource - Creates a new resource.
- PUT /resource/id - Updates an existing resource by its identifier.
- DELETE /resource/id - Deletes an existing resource by its identifier.
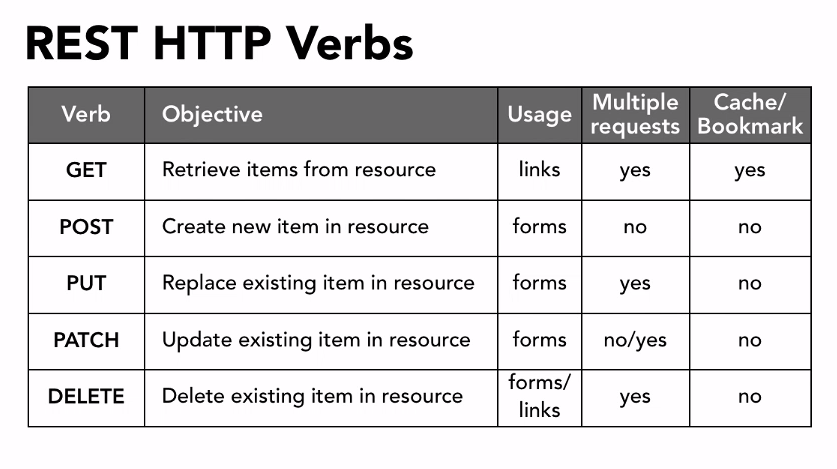
In Express.js, we can define these routes using the app object, which is an instance of the Express application. Here's an example of implementing RESTful routing in Express.js
const express = require('express'); const app = express();
// GET /users - Retrieve all users
app.get('/users', (req, res) => {
// Logic to retrieve all users
res.send('Retrieve all users');
});
// GET /users/:id - Retrieve a specific user
app.get('/users/:id', (req, res) => {
const userId = req.params.id;
// Logic to retrieve a user with the given id
res.send(Retrieve user with id ${userId}
);
});
app.post('/users', (req, res) => {
// Logic to create a new user using the data from req.body
res.send('Create a new user');
});
// PUT /users/:id - Update a specific user
app.put('/users/:id', (req, res) => {
const userId = req.params.id;
// Logic to update the user with the given id using the data from req.body
res.send(Update user with id ${userId}
);
});
// DELETE /users/:id - Delete a specific user
app.delete('/users/:id', (req, res) => {
const userId = req.params.id;
// Logic to delete the user with the given id
res.send(Delete user with id ${userId}
);
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Library
WEB DEVELOPMENT
Basic
Frontend
Backend
Node JS
Node Modules
Restful Routing
Static Files
REST API
Introduction to Node JS
GET vs POST
Database
Interview Questions
FAANG QUESTIONS
On this page
CRUD Operations
Restful Routes