Lists and Keys in React JS
Published by
sanya sanya
The lists and keys
are used to render dynamic sets of elements. When you have an array of data and you want to render each item in the array as a component, we can use a list in React.
To render a list of items in React, you typically use the map()
function on the array of data and return a component for each item. Each rendered component needs to have a unique identifier known as a "key."
The key is important because it helps React identify which items have changed, been added, or been removed when the list is updated.
The example of the working of the Lists and Keys
in React JS is mentioned below -
import React from 'react';
function MyListComponent({ items }) {
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
function App() {
const items = [
{ identity: 1, name: 'Item_1' },
{ identity: 2, name: 'Item_2' },
{ identity: 3, name: 'Item_3' }
];
return <MyListComponent items={items} />;
}
export default App;
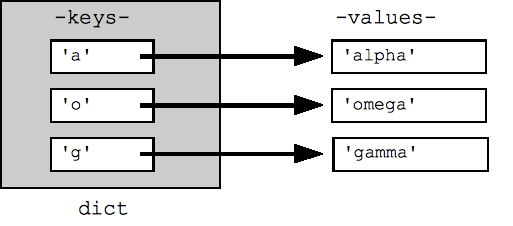
In the above example, the MyListComponent
receives an array of items as a prop. It uses the map()
function to iterate over each item in the array and render an "li" element for each item. The key={item.id} attribute assigns a unique key to each rendered "li" element, using the id property of each item.
The key should be a stable identifier and preferably unique among sibling elements. It helps React efficiently update the list by recognizing which items have changed or been rearranged. If the key is not provided or is not unique, React may encounter performance issues and potentially render incorrect results.
Library
WEB DEVELOPMENT
Basic
Frontend
Express JS
React
Hello World in ReactJS
Rendering Elements
States and Lifecycles in React JS
Handling Events in React JS
Introduction to JSX
Components and Props in React JS
Conditional Rendering
Lists and Keys in React JS
Introduction to Redux
Types of components (class vs functional)
Using the useEffect hook
Building custom hooks in React JS
Context API in React JS
Forms in React JS
Lifting State Up in React JS
Composition vs Inheritance
Prop drilling in React JS
Introduction to Hooks
Using the useState hook
UseMemo vs useCallback
Backend
Interview Questions
FAANG QUESTIONS