Lifting State Up in React JS
Published by
sanya sanya
"Lifting state up" is a common pattern in React where we move the state of a component higher in the component tree to make it accessible to multiple components that need to share and synchronize that state. This allows us to have a single source of truth for the state and ensure consistency across the application.
The example to illustrate the concept of lifting state up is mentioned below -
import React, { useState } from 'react';
function ChildComponent({ count, onIncrement }) {
return (
<div>
<p>Count: {count}</p>
<button onClick={onIncrement}>Increment</button>
</div>
);
}
function ParentComponent() {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
return (
<div>
<h2>Parent Component</h2>
<ChildComponent count={count} onIncrement={handleIncrement} />
</div>
);
}
function App() {
return <ParentComponent />;
}
export default App;
In the above example, the ParentComponent
holds the state variable count using the useState hook. It also defines the handleIncrement function, which updates the count by incrementing it.
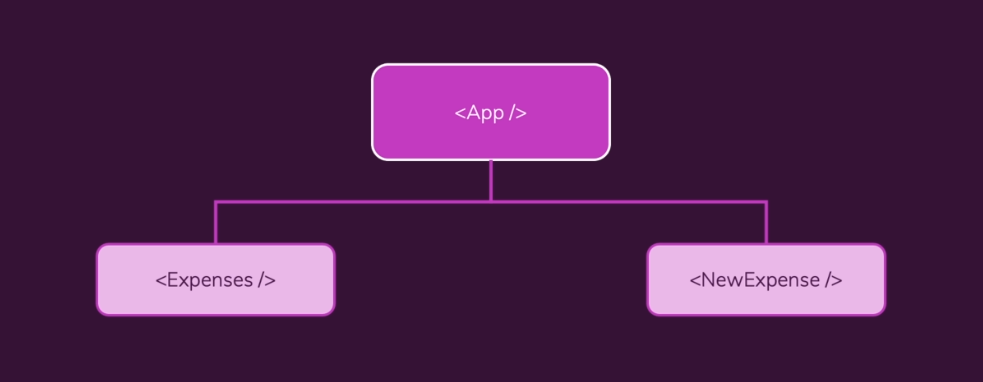
The count state and the handleIncrement function are passed as props to the ChildComponent
. The ChildComponent receives the count prop and displays it. When the button is clicked, the onIncrement prop (which is the handleIncrement function) is triggered, updating the state in the ParentComponent
.
By lifting the state up from ChildComponent
to ParentComponent, multiple child components can access and modify the same state. Any changes to the state in the ParentComponent will be automatically propagated to the child components, ensuring synchronization.
Lifting state up
is useful in scenarios where multiple components need to share and interact with the same data. It promotes data flow and maintainability by centralizing the state management and making it accessible to all the relevant components.
Library
WEB DEVELOPMENT
Basic
Frontend
Express JS
React
Hello World in ReactJS
Rendering Elements
States and Lifecycles in React JS
Handling Events in React JS
Introduction to JSX
Components and Props in React JS
Conditional Rendering
Lists and Keys in React JS
Introduction to Redux
Types of components (class vs functional)
Using the useEffect hook
Building custom hooks in React JS
Context API in React JS
Forms in React JS
Lifting State Up in React JS
Composition vs Inheritance
Prop drilling in React JS
Introduction to Hooks
Using the useState hook
UseMemo vs useCallback
Backend
Interview Questions
FAANG QUESTIONS