Introduction to JSX
Published by
sanya sanya
JSX
stands for JavaScript XML. It is a syntax extension for JavaScript that is commonly used in React.js
, a popular JavaScript library for building user interfaces. JSX
allows developers to write HTML-like code directly within JavaScript, making it easier to define and render user interface components.
With JSX
, we can create React elements by combining JavaScript expressions with HTML-like syntax. These elements can represent UI components, which can be rendered to the DOM (Document Object Model) or used as building blocks for more complex UI structures.
The example of JSX
Code in React JS
is mentioned below -
import React from 'react';
const App = () => {
const name = 'John Doe';
return (
<div>
<h1>Hello, {name}!</h1>
<p>Welcome to my React app.</p>
</div>
);
};
export default App;
In the above code, the App component is defined using a functional component syntax. Within the return statement, you can see how JSX
is used to define the structure and content of the component. The curly braces {} are used to embed JavaScript expressions, allowing you to dynamically insert values, such as the name variable in the example.
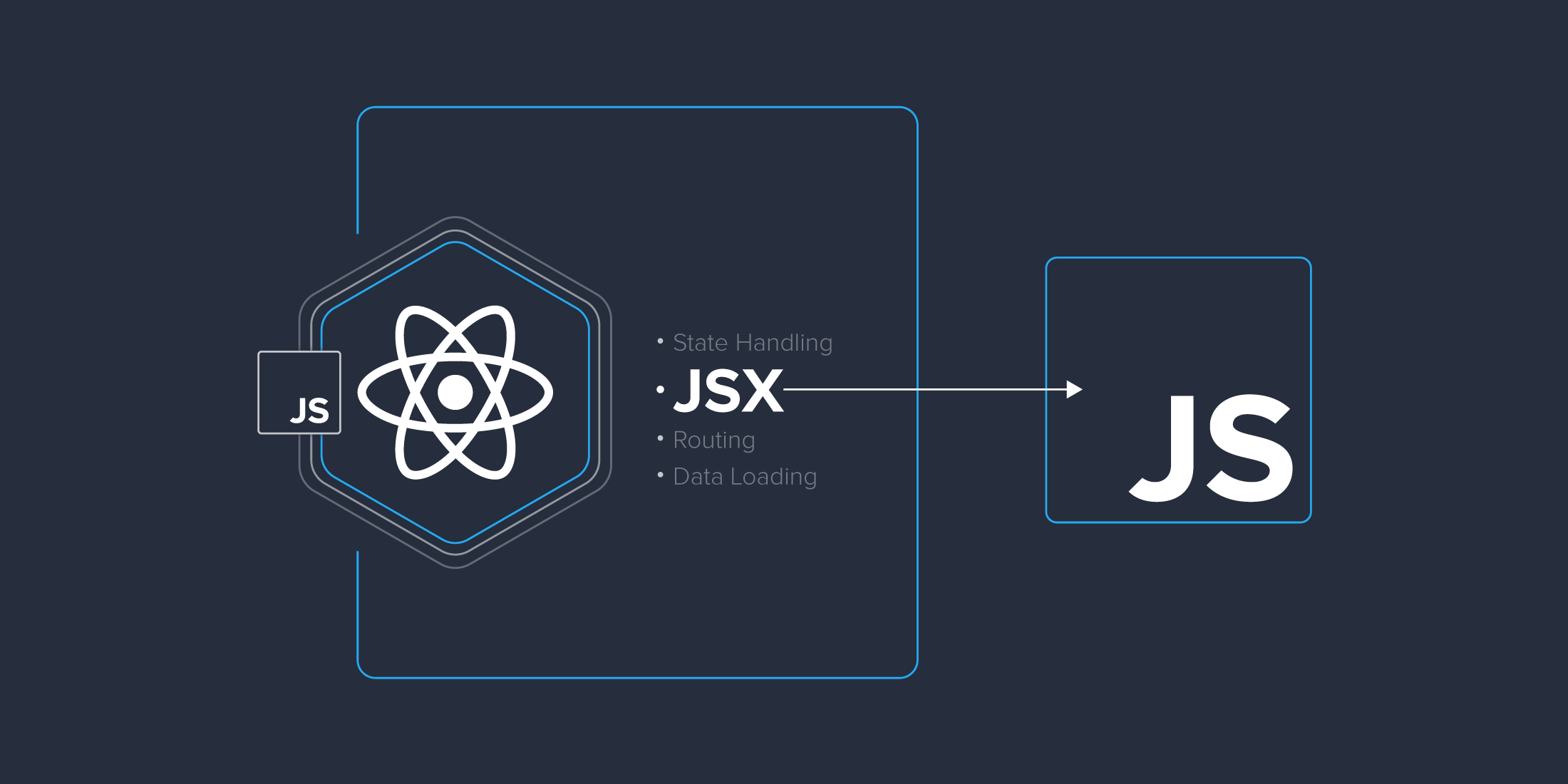
JSX
elements are transformed into React elements during the build process, using tools like Babel. The resulting React elements are then rendered to the DOM or used within other React components.
Library
WEB DEVELOPMENT
Basic
Frontend
Express JS
React
Hello World in ReactJS
Rendering Elements
States and Lifecycles in React JS
Handling Events in React JS
Introduction to JSX
Components and Props in React JS
Conditional Rendering
Lists and Keys in React JS
Introduction to Redux
Types of components (class vs functional)
Using the useEffect hook
Building custom hooks in React JS
Context API in React JS
Forms in React JS
Lifting State Up in React JS
Composition vs Inheritance
Prop drilling in React JS
Introduction to Hooks
Using the useState hook
UseMemo vs useCallback
Backend
Interview Questions
FAANG QUESTIONS