Hello World in ReactJS
Published by
sanya sanya
There are some steps which are necessary to follow to start coding in React JS
. The Points which are important to start with are mentioned below -
-
1 - Set Up the Development Environment - We must have to make sure that we have
Node.js
andnpm
(Node Package Manager) installed on our system. We can download them from the officialNode.js
website. -
2 - Create a React Project - Open the terminal or command prompt and navigate to the desired directory. Then, we have to run the command mentioned below to create a new
React project
.
npx create-react-app hello-world
-
3 - Navigate to the Project Directory - In this step, we have to move into the project directory by running the following command.
-
4 - Edit the App.js File - Open the src/App.js file in a text editor of your choice and replace its content with the following code.
import React from 'react';
function App() {
return (
<div>
<h1>Hello World!</h1>
</div>
);
}
export default App;
- 5 - Start the Development Server - To start the development server and see the "Hello World!" application in the browser, we have to run the following command.
npm start
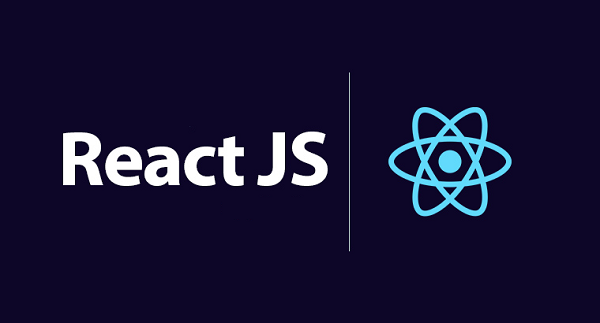
Library
WEB DEVELOPMENT
Basic
Frontend
Express JS
React
Hello World in ReactJS
Rendering Elements
States and Lifecycles in React JS
Handling Events in React JS
Introduction to JSX
Components and Props in React JS
Conditional Rendering
Lists and Keys in React JS
Introduction to Redux
Types of components (class vs functional)
Using the useEffect hook
Building custom hooks in React JS
Context API in React JS
Forms in React JS
Lifting State Up in React JS
Composition vs Inheritance
Prop drilling in React JS
Introduction to Hooks
Using the useState hook
UseMemo vs useCallback
Backend
Interview Questions
FAANG QUESTIONS