Handling Events in React JS
Published by
sanya sanya
Handling events
follows a similar pattern to handling events in regular HTML but with a few differences. Here's how we can handle events in React as mentioned below.
Event Binding
In the component's JSX
, we can attach event handlers by using the on[EventName] attribute, where [EventName] represents the name of the event we want to handle. For example, onClick, onSubmit, onChange, etc.
<button onClick={handleClick}>Click Me</button>
<input onChange={handleChange} />
<form onSubmit={handleSubmit}>
{/* form contents */}
</form>
Event Handler Functions
We need to define event handler functions that will be executed when the corresponding event occurs. These functions can be regular JavaScript functions or class methods. For instance,
function handleClick() { console.log('Button clicked!'); } function handleChange(event) { console.log('Input value changed:', event.target. value); } function handleSubmit(event) { event.preventDefault(); console.log('Form submitted!'); }
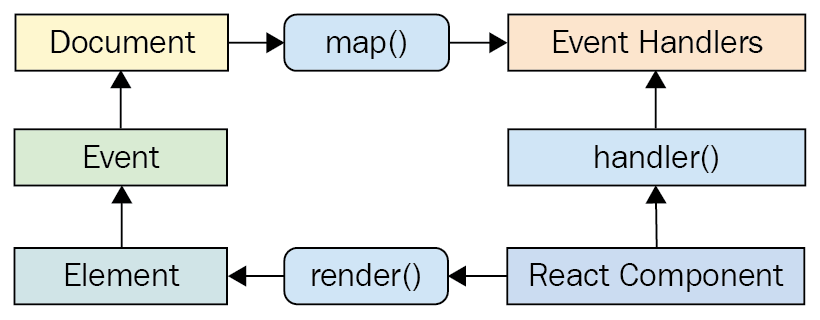
Updating State with Events
We can also use events to update the state of our components. To update the state, we should use the setState method provided by React. It is recommended to use the functional form of setState when the new state depends on the previous state. for example,
function App() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(prevCount => prevCount + 1);
}
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
}
In the example above, each time the button is clicked, the handleClick function is called, which updates the count state by incrementing its value.
Event Object
When an event occurs, React passes an event object as the first argument to the event handler function. This object contains information about the event, such as the target element and event-specific data.
function handleChange(event) {
console.log('Input value changed:', event.target. value);
}
In the handleChange function, event.target refers to the element that triggered the event (in this case, the input), and event.target.value gives us the current value of the input.
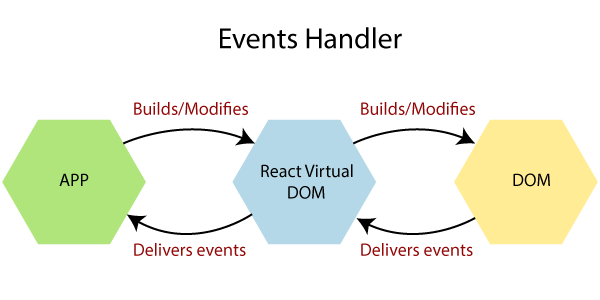
Remember to consider event binding and function references when passing event handlers to child components. We may need to bind the event handler using the bind method or use arrow functions to avoid incorrect function invocation or losing the correct this context.
Overall, handling events in React follows a familiar pattern, allowing us to create interactive and dynamic user interfaces.
Library
WEB DEVELOPMENT
Basic
Frontend
Express JS
React
Hello World in ReactJS
Rendering Elements
States and Lifecycles in React JS
Handling Events in React JS
Introduction to JSX
Components and Props in React JS
Conditional Rendering
Lists and Keys in React JS
Introduction to Redux
Types of components (class vs functional)
Using the useEffect hook
Building custom hooks in React JS
Context API in React JS
Forms in React JS
Lifting State Up in React JS
Composition vs Inheritance
Prop drilling in React JS
Introduction to Hooks
Using the useState hook
UseMemo vs useCallback
Backend
Interview Questions
FAANG QUESTIONS
On this page
Event Binding
Event Handler Functions
Updating State with Events
Event Object