Constructor Functions in JavaScript
Published by
sanya sanya
In JavaScript, constructor functions are used to create objects of the same type. They are a way of defining a blueprint for an object and then creating instances of that object.
Constructor functions are defined using the function keyword and are typically named with a capitalized first letter to distinguish them from regular functions.
The code showing the working of the Constructor Function is mentioned below -
function Person(name, age) { this.name = name; this.age = age; this.greet = function() { console.log(
Hello, my name is ${this.name} and I am ${this.age} years old.
); } } const person1 = new Person('Alice', 25); console.log(person1)
//Output: Person {name: 'Alice', age: 25, greet: ƒ}
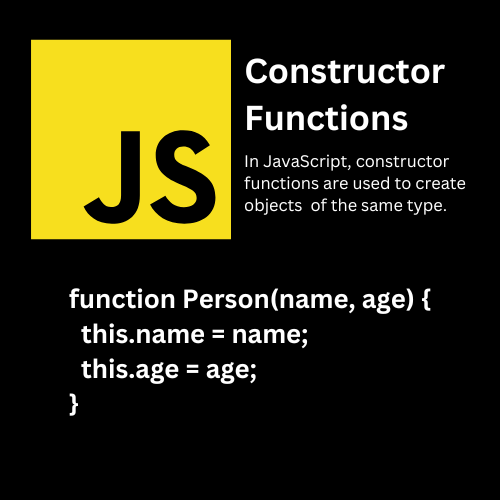
Sub-topics related to Constructor Function
Some Sub-topics related to Constructor Function are mentioned below -
- Prototypes - JavaScript uses prototypal inheritance, which means that objects can inherit properties and methods from other objects. This sub-topic covers how to use the prototype property to add methods and properties to objects and how to use inheritance in JavaScript.
- new Keyword - The new keyword is used to create a new instance of an object. This sub-topic covers how to use new to create new objects from constructor functions. The code showing the working of the new keyword is mentioned below -
// Constructor function for a person object function Person(name, age) { this.name = name; this.age = age; } // Creating a new instance of the person object using the
new
keyword const person1 = new Person('John', 30); console.log(person1.name); // Output: John console.log(person1.age); // Output: 30
- Constructor Chaining - Constructor functions can be chained together to create more complex objects. This sub-topic covers how to use constructor chaining to create objects that inherit from multiple parent objects.
// Parent object constructor function function Person(name, age) { this.name = name; this.age = age; }
// Child object constructor function function Employee(name, age, position) { Person.call(this, name, age); // Calling parent constructor function withcall
method this.position = position; }
// Creating a new instance of the Employee object const employee1 = new Employee('John', 30, 'Manager'); console.log(employee1.name); // Output: John console.log(employee1.age); // Output: 30 console.log(employee1.position); // Output: Manager
Library
WEB DEVELOPMENT
Basic
HTML - Hyper Text Markup Language
CSS - Cascading Style Sheets
JavaScript
An Introduction to Javascript!
How to Run JavaScript Code
Variables in Javascript
Numbers in JavaScript
JavaScript Operators
Data Types in JavaScript
Conditional Statements
Switch Statements
Loops in Javascript
Arrays in JavaScript
Strings in JavaScript
Objects in JavaScript
Object Methods in JavaScript
Functions in JavaScript
Object Referencing and Copying in JavaScript
' this' keyword
Asynchronous Programming in JavaScript
Callbacks in JavaScript
Promises in JavaScript
Constructor Functions in JavaScript
Async and Await in JavaScript
Type Conversion in Javascript
DOM
Currying in JavaScript
Network Request
Frontend
Backend
Interview Questions
FAANG QUESTIONS
On this page
Sub-topics related to Constructor Function