Components and Props in React JS
Published by
sanya sanya
In ReactJS, the components
are said to be the building blocks of user interfaces (UIs). They are reusable, self-contained pieces of code that encapsulate the logic and presentation of a part of the user interface. React uses a declarative syntax to describe how the UI should look based on the current state of the component.
There types of the Components in React JS in descriptive Manner are mentioned below.
Functional Components
Functional components
are written as JavaScript functions and are also known as stateless components. They receive props (short for properties) as arguments and return React elements to describe the UI.
Functional components are simpler and easier to read and test compared to class components. for instance,
import React from 'react';
const MyComponent = (props) => {
return <div>Hello, {props.name}!</div>;
};
export default MyComponent;
The functional components can now be stateful as well. Prior to hooks, only class components could have state using the this.state mechanism. Hooks were introduced in React version 16.8 to provide stateful and other React features to functional components without the need for class components.
Class Components
The Class components
are defined as ES6 classes and extend the base React.Component class. They have additional features like lifecycle methods and the ability to hold state.
Moreover, the Class components
are useful when you need to manage complex state or interact with the lifecycle of a component. for example,
import React from 'react';
class MyComponent extends React.Component {
render() {
return <div>Hello, {this.props.name}!</div>;
}
}
export default MyComponent;
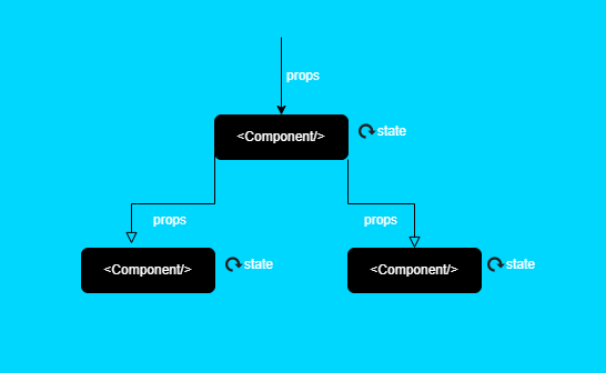
Props
Props
are used to pass data from a parent component to its child components. They are read-only and cannot be modified by the child components.
Props
help in creating reusable components that can be easily customized by providing different values for the props. for instance,
import React from 'react'; import MyComponent from './MyComponent';
const App = () => {
return <MyComponent name="John" />;
};
export default App;
In the above example, the name prop is passed to the MyComponent, and it can be accessed within the component using props.name
. The rendered output would be "Hello, John!".
Library
WEB DEVELOPMENT
Basic
Frontend
Express JS
React
Hello World in ReactJS
Rendering Elements
States and Lifecycles in React JS
Handling Events in React JS
Introduction to JSX
Components and Props in React JS
Conditional Rendering
Lists and Keys in React JS
Introduction to Redux
Types of components (class vs functional)
Using the useEffect hook
Building custom hooks in React JS
Context API in React JS
Forms in React JS
Lifting State Up in React JS
Composition vs Inheritance
Prop drilling in React JS
Introduction to Hooks
Using the useState hook
UseMemo vs useCallback
Backend
Interview Questions
FAANG QUESTIONS
On this page
Functional Components
Class Components
Props