Asynchronous Programming in JavaScript
Published by
sanya sanya
Asynchronous Programming is the technique in which the tasks are performed parallel without waiting for another task to be completed. Javascript
is Asynchronous Programming.
The concept of Asynchronous Programming
can be understood by simple programming. We have gone to several places such as Theatre, banks, Railway Stations, and so on.
But we have one thing in common there. Can't figure out what?... It is the queue or line of people at the ticket counter. Suppose two friends, Rakesh
and Mukesh
went to watch Fast X
and they have their show at 9 PM but they were quite late and went to the theatre at 8:50 PM. Where they figure out that the ticket counters are rushed and they have to wait for their turn in the line.
The system of ticket distribution is very slow because the person in the ticket window performs his tasks synchronously
which means he is giving one ticket and other people have to wait for their turn.
On the other hand, Naman
is a smart boy who doesn't like the last-minute hassle and he books his ticket on Bookmyshow
. So, the ticket booking at Bookmyshow is an example of Asynchronous
because various tasks can be performed at once and no one has to wait for their turn.
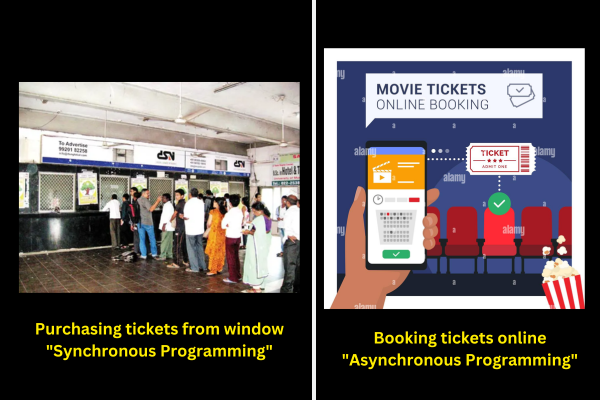
Why use Asynchronous Programming?
Asynchronous Programming has several uses because, in today's time where speed is the priority, this technique plays a vital role. However, some points why Asynchronous Programming
is used are mentioned below -
- Responsive User Interface - If an application has several buttons each having different functionality. If the user clicks a button, then the user still must have the privilege to click the other buttons too.
- Process Independent Data - The Application Programming Interface performs various calculations at the backend and sends the favorable outcome to the user without disturbing other executions.
- Scalability - When the server gets various system calls to maintain efficiency and scalability, Asynchronous Programming will be helpful.
Advantages of Asynchronous Programming Over Synchronous Programming
Several Advantages of Asynchronous Programming Over Synchronous Programming are available which makes Asynchronous Programming more advantageous to use. Some of the advantages are mentioned below -
- `Async` is multithreaded whereas `Sync` is single-threaded.
- `Async` is non-blocking whereas `Sync` is blocking.
- Asynchronous Programming is faster whereas Synchronous Programming is Slower.
- Throughput is high in Asynchronous Programming whereas throughput is low in Synchronous Programming
Callback Function
The Callback
function is the function that is executed after the execution of the first function.
For instance, Suman
has a party in her house and she makes a variety of dishes for guests. However, she prepares only one variety of dessert which is to be served after the main course.
Suman told her maid to only serve the sweet dish after all the guests has finished their main course food. Suman's Maid
said her to call her "Main course finished" and after that, she will serve the sweet dish.
In the example, the main function which will execute first will be "Main Course Completion" and the callback function which will execute after the first function execution is "Dessert serving".
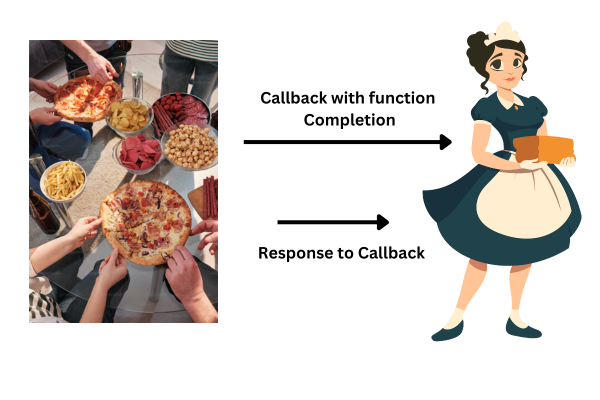
function dataFetch(callback) { setTimeout(() => { const data = {name: "Pathaan", age: 50}; callback(data); }, 2000); } //Function execution with callback dataFetch(function(data) { console.log(data); }); console.log("Data is being fetched...");
//Output: Data is being fetched... //After two seconds {name: 'Pathaan', age: 50}
Promises
The Promises
in Javascript is a function that represents a way of handling async operations in an organized manner. It also acts as a placeholder for a future value.
The syntax of the Promise in Javascript is mentioned below -
const promise = new Promise(function(resolve, reject){});
Library
WEB DEVELOPMENT
Basic
HTML - Hyper Text Markup Language
CSS - Cascading Style Sheets
JavaScript
An Introduction to Javascript!
How to Run JavaScript Code
Variables in Javascript
Numbers in JavaScript
JavaScript Operators
Data Types in JavaScript
Conditional Statements
Switch Statements
Loops in Javascript
Arrays in JavaScript
Strings in JavaScript
Objects in JavaScript
Object Methods in JavaScript
Functions in JavaScript
Object Referencing and Copying in JavaScript
' this' keyword
Asynchronous Programming in JavaScript
Callbacks in JavaScript
Promises in JavaScript
Constructor Functions in JavaScript
Async and Await in JavaScript
Type Conversion in Javascript
DOM
Currying in JavaScript
Network Request
Frontend
Backend
Interview Questions
FAANG QUESTIONS
On this page
Why use Asynchronous Programming?
Advantages of Asynchronous Programming Over Synchronous Programming
Callback Function
Promises