Arrays in JavaScript
Published by
sanya sanya
We have variables to store the values. But when we have to store thousands of values, we have to make thousands of variables which will make the code much more hectic. So, the solution for such a case is "Array"
.
Array
is a linear data structure
which is used to store multiple values of the same type. One array
can store multiple values.
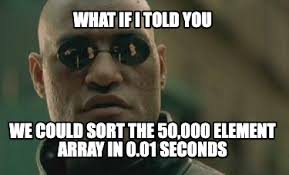
The methods for creating arrays are as follows -
const arr = ["one", "two", "three"] const arr = new Array ("one", "two", "three")
Common Operations on Arrays
Some operations can be performed on arrays such as -
- Accessing Array Elements - The elements in the array are accessed through the code below
- Changing an Array Element - The Elements in the `Array` can be changed by the code below -
- Objects and Arrays - The type of `arrays` in Javascript is an object. The code showing the same is as follows -
- Looping Array Elements - The code for `Looping through an array` is mentioned below -
- Adding Array Elements - The code for appending the new values to the `Array` is mentioned below -
const arr = ["one", "two", "three"] console.log(arr[1])
//Output: two
const arr = ["one", "two", "three"] arr[0] = "four" console.log(arr)
//Output: four two three
const arr = ["one", 1] console.log(arr) //Output: one 1
const arr = ["one", "two", "three", "four"] for(let i = 0; i < arr.length; i++) { console.log(arr[i]) }
//Output: One Two Three Four
const arr = ["one", "two", "three"]; arr.push("five"); console.log(arr);
//Output: ['one', 'two', 'three', 'five']
Array Methods
Several Methods are supported by Arrays
in Javascript and each Array Method
is mentioned below -
- ToString()- The Javascript `toString()` method is used to convert an array to comma-separated strings. The code for the same is as follows -
- Join() - The `join()` method is used to join all the elements in the array. The code for the same is as follows -
- Pop() - The `pop()` method is used to remove the last element from an array. The code for the same is as follows -
- Push() - The Javascript `push()` method is used to add a new element at the end of the array. Also, it returns the new array length. The code for the same is as follows -
- Shift() - The
shift()
removes the first element from the array and moves all the other elements to the lower index. The code for the same is as follows - - Unshift() - The
unshift()
method is used to add an element at the beginning of an array and reorder older elements. The code for the same is as follows - - Deleting Array Elements - The Elements in the arrays can be deleted as shown in the syntax below -
- Arrays Concatenation -The
concat()
method is used to concate two arrays as mentioned below - - Array Splicing and Slicing - The
Slice()
method is used to add new items to an array whereas theSplice()
method is used to fetch a slice of an array. The code for both is as follows - - Array Sorting -
Arrays
Support a method to sort the elements. The code for the same is as follows - - Arrays Iteration with forEach() - We can also use Array.forEach() function to iterate through an array. The working is initiated through the code below -
- Array Split - The split() method is used to split the string into an array as per the given delimiters. For instance,
- Array length - This property is used to find the length of an array. The code for the same is as follows -
- Array Reverse - This method is used to return the reverse of an input array. The code for the same is as follows -
- find - The find function is used to find an element based on the specific condition. The search will stop when it returns true and if an item or element doesn't found, the result will be undefined.
- findIndex - This returns the index where the element of the item was found instead of the element itself. The code showing the working of `findIndex` is mentioned below -
- findLastIndex - It have the same working as of the findIndex. However, it starts searching from left to right. The code for the same is as follows -
const arr = ["one", "two", "three"]; console.log(arr.toString(",")); //Output:
One, two, three
const arr = ["one", "two", "three"] console.log(arr.join("-"))
//Output: one-two-three
const arr = ["one", "two", "three"] arr.pop();
//Output: three
const arr = ["one", "two", "three"] console.log(arr.push("four"))
//Output: one two three four
const arr = ["one", "two", "three"] console.log(arr.shift())
//Output: one
const arr = ["one", "two", "three"] console.log(arr.unshift("four"))
//Output: four one two three
const arr = ["one", "two", "three"] delete arr[1]
const arr_1 = ["one", "two"] const arr_2 = ["three", "four"] const arr_3 = arr_1.concat(arr_2) console.log(arr_3)
//Output: ['one', 'two', 'three', 'four']
Array Splice(): const arr = ["one", "two", "three", "four"] arr.splice(2,2,"five","six")
//Output: ["three", "four"] Will return the removed values
Array Slice():
const arr = ["one", "two", "three", "four"]
const arr_2 = arr.slice(1,3)
console.log(arr_2)
//Output:
['two', 'three']
const arr = [6, 1, 4, 7, 2, 9]
console.log(arr.sort())
//Output: [1, 2, 4, 6, 7, 9]
const numbers = ['one', 'two', 'three']; function iter_num(item) { console.log(item); } numbers.forEach(iter_num);
//Output: one two three
let numbers = 'one, two, three'; let arr = numbers.split(', '); for (let numbers of arr) { console.log(
A message to ${numbers}.
); }
//Output: A message to one. A message to two. A message to three.
const arr = [6, 1, 4, 7, 2, 9] console.log(arr.length)
//Output: 6
const arr = [6, 1, 4, 7, 2, 9] console.log(arr.reverse())
Output: [9, 2, 7, 4, 1, 6]
The code for the same is mentioned below -
let users = [ {id: 01, name: "Pathan"}, {id: 02, name: "Tiger"}, {id: 03, name: "Kabeer"} ]; let user = users.find(item => item.id == 1); console.log(user.name);
//Output: Pathan
let users = [ {id: 01, name: "Pathan"}, {id: 02, name: "Tiger"}, {id: 03, name: "Kabeer"} ]; console.log(users.findIndex(user => user.name == 'Tiger'));
//Output: 1
It will return -1 if nothing is found.
let users = [ {id: 01, name: "Pathan"}, {id: 02, name: "Tiger"}, {id: 03, name: "Kabeer"} ]; console.log(users.findLastIndex(user => user.name == 'Kabeer'))
//Output: 2
Arrays Map, Filter, and Reduce
There are three different methods map
, filter
, and reduce
. The detailed description for all three is mentioned below.
Map()
The Map()
function doesn't change the original array however it performs operations on each array element and creates a new array. The code for the same is mentioned below.
const num_1 = [45, 4, 9, 16, 25]; const num_2 = num_1.map(myFunction); function func(value, index, array) { return value * 2; }
//Output: Each element in the array will be multiplied by 2 and the final output will be - [90,8,18,32,50]
Filter()
The filter()
method returns a new array after performing a test on each array. The elements which passed the test will be returned in the output as mentioned in the code below -
const num = [45, 4, 9, 16, 25]; const result = num.filter(func); function func(value, index, array) { return value > 18; } //Output: The values which are greater than 18 are returned: 25, 45
Reduce()
The reduce()
function in javascript runs a function over the array elements to reduce them to a single unit.
Moreover, the array.reduce and array.reduceRight functions are used to calculate a single value based on the array.
The syntax for the same is as follows -
let value = arr.reduce(function(accumulator, item, index, array) { // ... }, [initial]);
The terms which are mentioned in the syntax are mentioned below -
- The accumulator is the result of the previous function calls.
- item is the current item on which the operation is being performed.
- index is the position where the particular element gets stored.
- array represents the array.
const num = [45, 4, 9, 16, 25]; let sum = num.reduce(func); function func(total, value, index, array) { return total + value; } //Output: The sum of all the elements will return 99
Library
WEB DEVELOPMENT
Basic
HTML - Hyper Text Markup Language
CSS - Cascading Style Sheets
JavaScript
An Introduction to Javascript!
How to Run JavaScript Code
Variables in Javascript
Numbers in JavaScript
JavaScript Operators
Data Types in JavaScript
Conditional Statements
Switch Statements
Loops in Javascript
Arrays in JavaScript
Strings in JavaScript
Objects in JavaScript
Object Methods in JavaScript
Functions in JavaScript
Object Referencing and Copying in JavaScript
' this' keyword
Asynchronous Programming in JavaScript
Callbacks in JavaScript
Promises in JavaScript
Constructor Functions in JavaScript
Async and Await in JavaScript
Type Conversion in Javascript
DOM
Currying in JavaScript
Network Request
Frontend
Backend
Interview Questions
FAANG QUESTIONS
On this page
Common Operations on Arrays
Array Methods
Arrays Map, Filter, and Reduce
Map()
Filter()
Reduce()